클래스와 접근 제어자
- -
안녕하세요 lika-7입니다
이번 시간에는 TypeScript의 클래스와 접근제어자에 대해 정리하겠습니다
클래스 기본 문법
//TypeScript
class UserA {
constructor(first:string, last:string, age:number){
this.first = first
this.last = last
this.age = age
}
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
클래스를 선언하는 위의 문법은 자바스크립트 문법에서는 문제가 없습니다.
하지만 타입스크립트에서 위와 같이 클래스를 선언하면 문제가 생깁니다
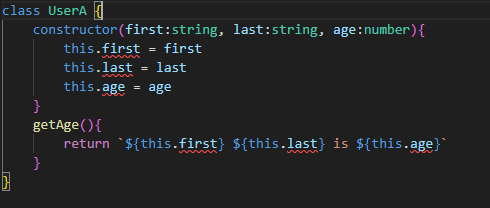

UserA안에 first의 내용이 없다고 나옵니다. last와 age 또한 마찬가지 입니다
//TypeScript
class UserA {
first: string
last: string
age: number
constructor(first:string, last:string, age:number){
this.first = first
this.last = last
this.age = age
}
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
constructor 윗부분에 생성자 함수의 속성 타입을 정의해주면 코드상 에러가 사라집니다
클래스 초기화
//TypeScript
class UserA {
first: string = ''
last: string = ''
age: number = 0
constructor(first:string, last:string, age:number){
this.first = first
this.last = last
this.age = age
}
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
first: string = ''
last: string = ''
age: number = 0
초기화가 필요하면 “=” 연산자를 이용하여 초기화 하면 됩니다.
접근 제어자 Access modifier
public 제어자
//TypeScript
class UserA {
first: string = ''
last: string = ''
age: number = 0
constructor(first:string, last:string, age:number){
this.first = first
this.last = last
this.age = age
}
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
위 코드에서
first: string = ''
last: string = ''
age: number = 0
부분에 public이라는 접근 제어자를 붙여도 같은 내용 입니다
public first: string = ''
public last: string = ''
public age: number = 0
위와 같이 public 접근 제어자를 넣는다면
//TypeScript
class UserA {
public first: string = ''
public last: string = ''
public age: number = 0
constructor(first:string, last:string, age:number){
this.first = first
this.last = last
this.age = age
}
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
class UserB extends UserA{
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
class UserC extends UserB{
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
const neo = new UserA('Neo','Anderson', 102)
console.log(neo.first)
console.log(neo.last)
console.log(neo.age)
위의 코드에서
protected
//TypeScript
class UserA {
public first: string = ''
protected last: string = ''
public age: number = 0
constructor(first:string, last:string, age:number){
this.first = first
this.last = last
this.age = age
}
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
class UserB extends UserA{
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
class UserC extends UserB{
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
const neo = new UserA('Neo','Anderson', 102)
console.log(neo.first)
console.log(neo.last)
console.log(neo.age)
protected last: string = ''
UserA 클래스의 last 속성을 protected로 접근제어 한다면
class UserB extends UserA{
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
class UserC extends UserB{
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
파생 클래스인 UserB,UserC 는 UserA의 last 속성에 접근이 가능하니 문제가 생기지 않지만
const neo = new UserA('Neo','Anderson', 102)
console.log(neo.first)
console.log(neo.last)
console.log(neo.age)
인스턴스의 부분인 console.log(neo.last) 부분에서 에러가 생깁니다

last 속성은 proteced 되어 있어 UserA와 그 서브 클래스에서만 접근 가능하다고 나옵니다
private
//TypeScript
class UserA {
public first: string = ''
protected last: string = ''
private age: number = 0
constructor(first:string, last:string, age:number){
this.first = first
this.last = last
this.age = age
}
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
class UserB extends UserA{
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
class UserC extends UserB{
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
const neo = new UserA('Neo','Anderson', 102)
console.log(neo.first)
console.log(neo.last)
console.log(neo.age)
private age: number = 0
private로 선언된 age는 UserA 내에서만 접근 가능하고
class UserB extends UserA{
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
class UserC extends UserB{
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
const neo = new UserA('Neo','Anderson', 102)
console.log(neo.first)
console.log(neo.last)
console.log(neo.age)
UserB, UserC 클래스와 neo 인스턴스에서는 접근이 불가능 합니다

age 속성은 private 임으로 UserA 클래스에서만 접근이 가능하다고 합니다
class UserB extends UserA{
protected getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
접근 제어자 - 그 외 패턴
//TypeScript
class UserA {
public first: string = ''
protected last: string = ''
private age: number = 0
constructor(first:string, last:string, age:number){
this.first = first
this.last = last
this.age = age
}
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
위의 내용을 보았을 때 생성자 선언에 똑같은 내용이 너무 많아서 보기 불편합니다.
- “속성”을 만들고 “속성”을 초기화 하고
- 생성자 안에서 같은 “속성”을 매개변수로 받아서
- 생성자 “속성”을 클래스의 속성으로 초기화 한다
똑같은 “속성”을 총 3번이나 작성하였습니다. 이를 아래와 같이 수정 할 수 있습니다
//TypeScript
class UserA {
constructor(public first:string = '', protected last:string = '', private age:number = 0){
}
getAge(){
return `${this.first} ${this.last} is ${this.age}`
}
}
'프론트엔드 > typescript' 카테고리의 다른 글
제네릭(Generic) -클래스 (0) | 2023.09.20 |
---|---|
제네릭(Generic) -함수 (0) | 2023.09.20 |
함수 - 오버로딩 (0) | 2023.09.19 |
함수 - 명시적 this 타입 (0) | 2023.09.19 |
객체 데이터 타입 지정 (0) | 2023.09.19 |
소중한 공감 감사합니다